Integrating Libraries into Your Vue.js Framework Projects
- Proper Toons
- Dec 3, 2024
- 5 min read
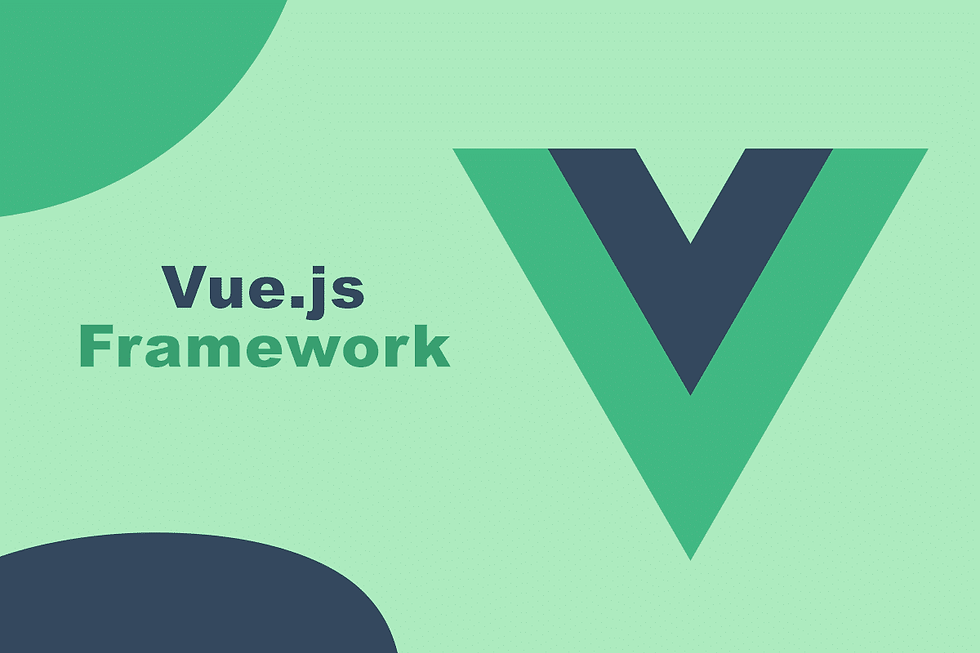
Vue.js has gained significant popularity among web developers due to its simplicity, reactivity, and component-based architecture. While Vue.js provides a rich set of features out of the box, integrating external libraries into your Vue.js projects can further extend its capabilities and help you build complex, dynamic applications more efficiently.
This article offers in-depth insights into how to effectively integrate libraries into your Vue.js framework projects, along with practical steps, tips, and best practices. Whether you’re working on a small-scale project or a large, enterprise-level application, this guide will provide valuable strategies to enhance the flexibility, performance, and maintainability of your codebase.
Why Integrating Libraries in Vue.js Is Beneficial
Before diving into the process of integration, it's essential to understand why you might want to use external libraries in the first place. Libraries can help solve common challenges or add advanced functionality to your Vue.js project, without reinventing the wheel. Here are a few reasons why integrating libraries makes sense:
Time-saving: Libraries provide pre-built solutions, enabling you to focus on business logic and other unique aspects of your application.
Feature Enhancement: Some libraries offer features that are not part of Vue.js by default, such as advanced charts, state management, or UI components.
Community Support: Many libraries are backed by active communities, providing regular updates, bug fixes, and enhancements.
Compatibility: Vue.js is compatible with many modern JavaScript libraries, making it easier to integrate them into your projects.
Key Considerations Before Integration
When integrating libraries into your Vue.js framework projects, certain considerations must be taken into account to ensure smooth implementation and long-term maintainability. Let’s explore some of these considerations in detail:
1. Library Compatibility
The first step in integrating a library is confirming that it is compatible with the version of Vue.js you're using. While Vue.js is highly flexible, not all third-party libraries are built with Vue in mind. Some libraries may require special wrappers or modifications to work seamlessly with Vue.js.
Make sure to check:
The library’s documentation for Vue.js compatibility.
Whether the library has a dedicated Vue.js wrapper (for example, Vuex for state management or Vue Router for navigation).
The community feedback on GitHub or other development forums regarding its compatibility with Vue.js.
2. Library Size and Performance
While external libraries offer extended functionality, many of them come with a significant performance overhead. It’s crucial to assess the size of the library and its potential impact on page load time.
Tools like Webpack Bundle Analyzer and Lighthouse can help you evaluate the bundle size and overall performance after integrating a new library. It’s a good practice to use tree-shaking (removing unused code) and code-splitting (splitting the application into smaller chunks) techniques to optimize performance.
3. Vue.js Ecosystem Integration
Vue.js has a well-established ecosystem that includes Vuex (for state management), Vue Router (for routing), and Vue CLI (for project setup). When integrating external libraries, make sure that they align with the Vue ecosystem’s principles, ensuring a seamless integration and avoiding potential conflicts.
For example, if you are integrating a third-party form validation library, check if it works well with Vue’s reactive data binding system. Libraries that follow Vue’s core principles will be easier to maintain and update as part of your Vue project.
How to Integrate Libraries into Your Vue.js Project
Once you’ve identified a suitable library for your project, the next step is integration. Here’s a step-by-step approach to integrating external libraries into your Vue.js framework projects.
Step 1: Install the Library
Most libraries can be installed via npm or yarn. Begin by installing the library using one of these commands in your project’s root directory.
npm install library-name OR yarn add library-name
This will add the library to your package.json file and allow you to import it into your project.
Step 2: Import the Library into Your Vue Component
Once the library is installed, the next step is importing it into your Vue component. Depending on how the library is structured, you can either import it globally or locally.
Local Import Example:
If you want to import the library into a specific Vue component, you can do so like this:
<script>
import Library from 'library-name';
export default {
data() {
return {
libraryInstance: new Library(),
};
},
};
</script>
Global Import Example:
If you need to use the library throughout your entire application, you can import it globally by modifying your main.js or app.js file:
import Vue from 'vue';
import Library from 'library-name';
Vue.use(Library);
This makes the library available across all components in the project.
Step 3: Utilize the Library Features
Now that the library is integrated, start utilizing its features within your components. For example, if you’re integrating a charting library, you can pass in data and customize the charts as follows:
<template>
<div>
<chart :data="chartData" :options="chartOptions" />
</div>
</template>
<script>
import ChartLibrary from 'chart-library';
export default {
data() {
return {
chartData: [/* some data */],
chartOptions: { /* options for the chart */ },
};
},
components: {
Chart: ChartLibrary,
},
};
</script>
Step 4: Handle Dependency and Conflict Management
Some libraries might have dependencies of their own, which could conflict with existing packages in your project. Make sure to carefully read the documentation for any setup instructions. Vue.js plugins or libraries that manipulate the DOM (like jQuery) might require special attention to ensure compatibility with Vue’s virtual DOM and reactivity system.
You can use Vue’s provide/inject API to share state or methods across components, or leverage mixins and directives to customize behavior.
Choosing the Right Web Design Company in USA
When working with external libraries, particularly those that enhance the user interface or involve custom styles, collaborating with an experienced web design company in USA can make a significant difference. These companies have a deep understanding of Vue.js and related technologies, ensuring that libraries integrate smoothly while providing an aesthetically pleasing user experience.
For instance, hiring a Venture Web Designer or any other reliable company can help ensure that your project benefits from expert-level integration, clean code practices, and seamless user interactions. With the right team, integrating libraries will not only be efficient but also elevate the overall quality of your application.
Best Practices for Managing Integrated Libraries
As your project grows, managing multiple libraries becomes crucial. Here are some best practices for managing libraries effectively:
Version Control: Ensure that all libraries are up-to-date, and use npm-check or similar tools to manage outdated dependencies.
Modularization: Break your application into smaller, more manageable modules so that libraries can be included only when needed.
Documentation: Keep detailed documentation of the libraries used, including installation instructions, configuration details, and usage guidelines for your team.
Testing: Always test the integration of new libraries in isolated environments before rolling them out in production.
Conclusion
Integrating external libraries into your Vue.js framework projects is a powerful way to add new features, improve functionality, and streamline your development process. By following best practices for compatibility, performance optimization, and ecosystem integration, you can ensure that your project remains scalable and maintainable. Always be mindful of the libraries you choose, as well as how they are integrated, to avoid potential pitfalls in long-term development.
Bình luận